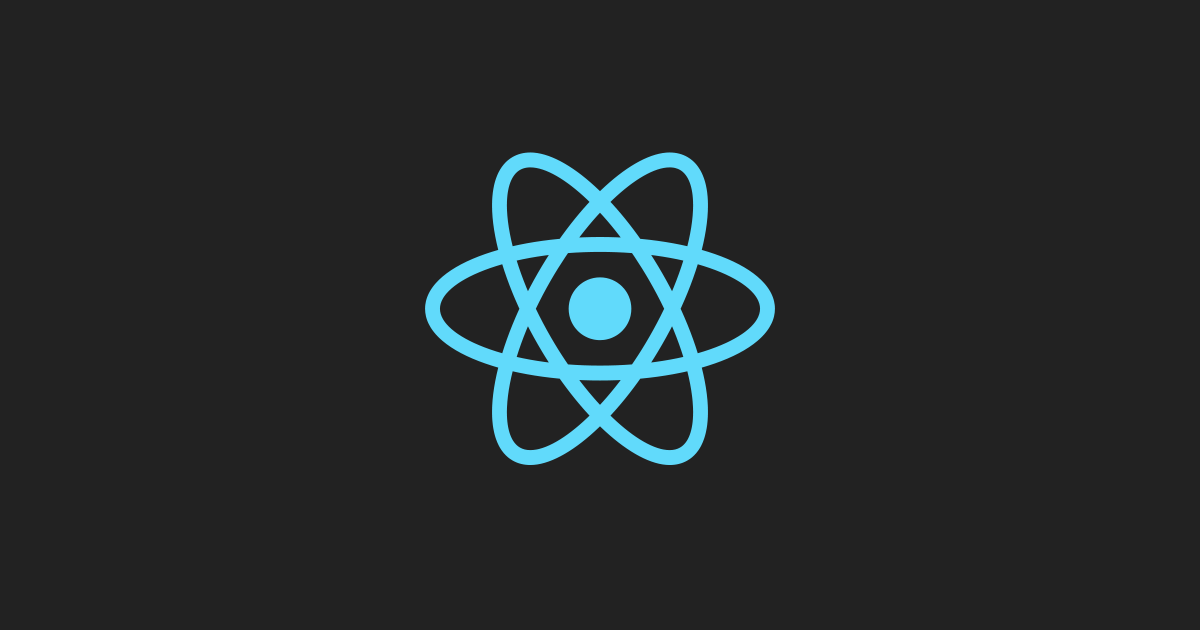
Use redux with react
2016, Jul 24
package
$ npm install react-route redux react-router-redux (works better with react-router)
#action A plain javascript object. Flux Standard Action could be the definition of action crossing app.
// define action name into a constants
import ActionNames from "../constants/actions"
let action = {
type: ActionsNames.DOSOMTHING
payload: {
// a json friendly javascript plain object.
},
error: true, // indicate the play is the error object.
meta: meta information relate to payload, but not a payload.
}
Utility Function
npm install flux-standard-action
import { isFSA } from 'flux-standard-action';
reducer
Do not change state, should alwasy return new if require change. Do not call api or Date.Now style function which is part of action
connect in react
connect([mapStateToProps], [mapDispatchToProps], [mergeProps], [options])
function mapStateToProps(state) {
return {
number: state.number // bind store value to react props
}
}
//bind action with (args) => dispatch(action(args));
function mapDispatchToProps() {
}
Notes: if don’t provide mapDispatchToProps, should dispath action with
this.props.dispatch(someActionCreator('arg'))
#A sample how to use
function mapStateToProps(state) {
return {
propName: state.propName
};
}
function mapDispatchProps(dispatch) {
return {
someAction: (arg) => dispatch(someActionCreator(arg)),
otherActions: bindActionCreators(actionCreators, dispatch)
};
}
class App extends Component {
render() {
// `mapStateToProps` 和 `mapDispatchProps` 返回的字段都是 `props`
const { propName, someAction, otherActions } = this.props;
return (
<div onClick={someAction.bind(this, 'arg')}>
{propName}
</div>
);
}
}
export default connect(mapStateToProps, mapDispatchProps)(App);
// Another way
connect(
state => {
number: state.number
},
{increase, descrease}
)(App);